New to Vue.js? Let's talk about how to create custom directives.
Vue has some default directives, like v-for, v-if, and v-on. Vue also allows you to create custom directives to alter data from some selected element and create a good shared code. This article will give a basic explanation of how to create custom directives using Vue.js.
Creating Custom Directives
Directives can be considered as an extension of html; they allow you to add a behavior to the element. As mentioned above, the directive's code can be shared with other html elements or components.
Directives in Vue can be created using Vue.directive(‘directive-name’, {}). The directive is similar to a component, where the first parameter is the directive identifier and the second is the object:
Note that our directive uses the prefix ‘v’. Every directive needs this prefix, because without it, Vue cannot identify a directive.
Custom directives also have some hooks that we can use:
-
bind - Called once when attached to the element.
-
inserted - Called when the element is inserted into the parent node.
-
update - Called after bind, when the initial value is modified. The new value becomes the argument.
-
unbind - Called once when the policy is unlinked from the element.
All these hooks are optional. Below, we'll give an example of a custom directive structure with some hook functions:
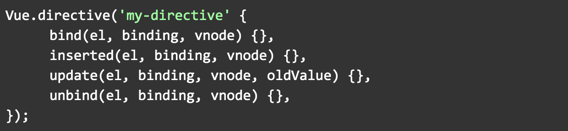
In our example, we will use the hooks bind and inserted, with el and binding objects. These objects have some arguments that we use more than others, and these include value and arg. (If you want to know more about arguments, I recommended reading about Directive Hook Arguments.)
Custom Directive Example
Now that we have an overview of how to create custom directives and hook functions, we will provide an example by creating a custom directive that changes a text color.
In the last example, the color is fixed, but sometimes, you can add colors dynamically, as follows:
In our next example, the directive is receiving an object with positions to align the text.
Custom directives can also receive arguments. Let's change our last code and add some arguments.
Now our directive is receiving dynamic arguments.
Components with Directives
All directives that were created before are considered global directives, meaning they could be accessed in every component in an application.
Custom directives created inside a component, however, are locally directive, meaning they can only be used within the component in which they are registered.
Note that the input created outside the directive doesn’t receive the focus behavior.
Conclusion
As shown above, custom directives are easy to create and quite simple to understand. Custom directives can help us create shared and reusable code. Sometimes, we need to create simple code that can be used in many components, and custom directives can help with this.
If you want to know more about custom directives, I recommended reading about Directives In Depth.
Happy coding!!!
Author
Eduardo Lucas Paoli
Eduardo Lucas Paoli is a UI Engineer at Avenue Code.